Unlocking the world of embedded software engineering requires a well-crafted roadmap. In this article, we will guide you through the essential steps to embark on a thrilling journey towards becoming an accomplished embedded software engineer. From gaining the necessary skills to exploring the vast realm of possibilities, join us as we unveil the path to success in this captivating field.
The Role of Embedded Software Engineers
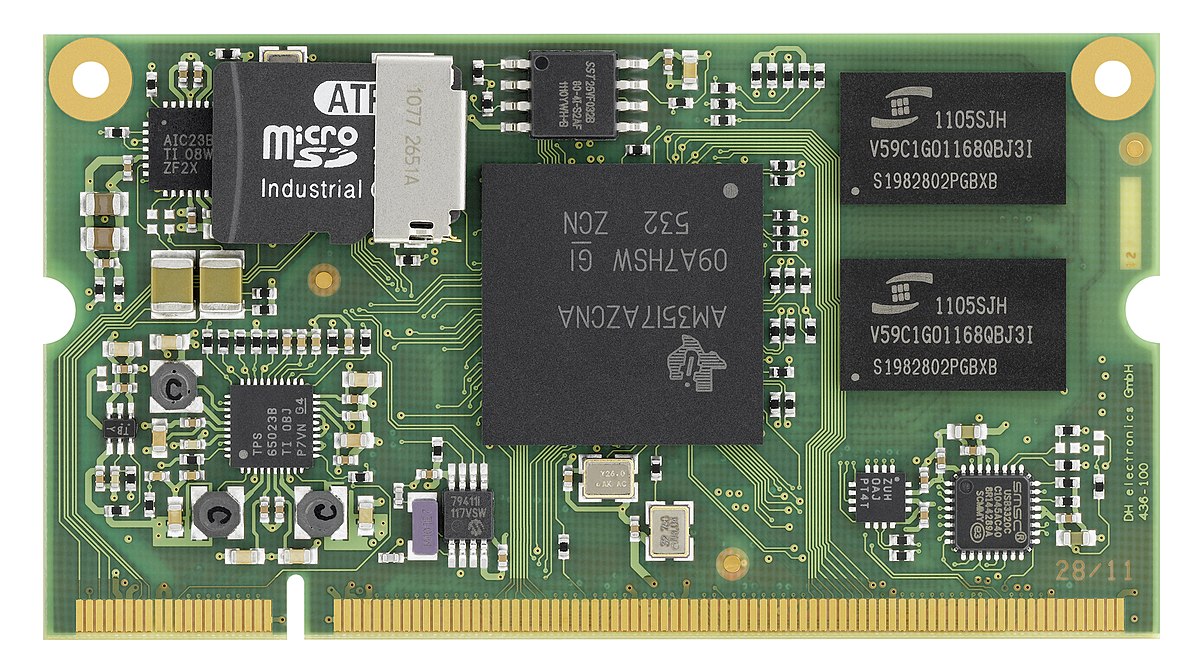
Embedded software engineers need a strong background in computer engineering or electrical engineering, and they must be proficient in programming languages such as C and C++. They also need to have a solid understanding of software engineering principles and methodologies.
To become an embedded software engineer, it is recommended to start by gaining a strong foundation in computer science or engineering through formal education or self-learning. Learning programming languages like C and C++ is essential, as well as familiarizing oneself with various development tools and platforms.
Hands-on experience is crucial in this field, so it is important to work on projects that involve programming microcontrollers, interfacing with various hardware components, and debugging software bugs. This can be done through internships, personal projects, or working with startup companies.
Continuous learning and staying updated with the latest advancements in embedded systems is also important for embedded software engineers. This can be achieved by attending workshops, conferences, and taking online courses or Linux training.
Meeting the Demand for Embedded Software Engineers
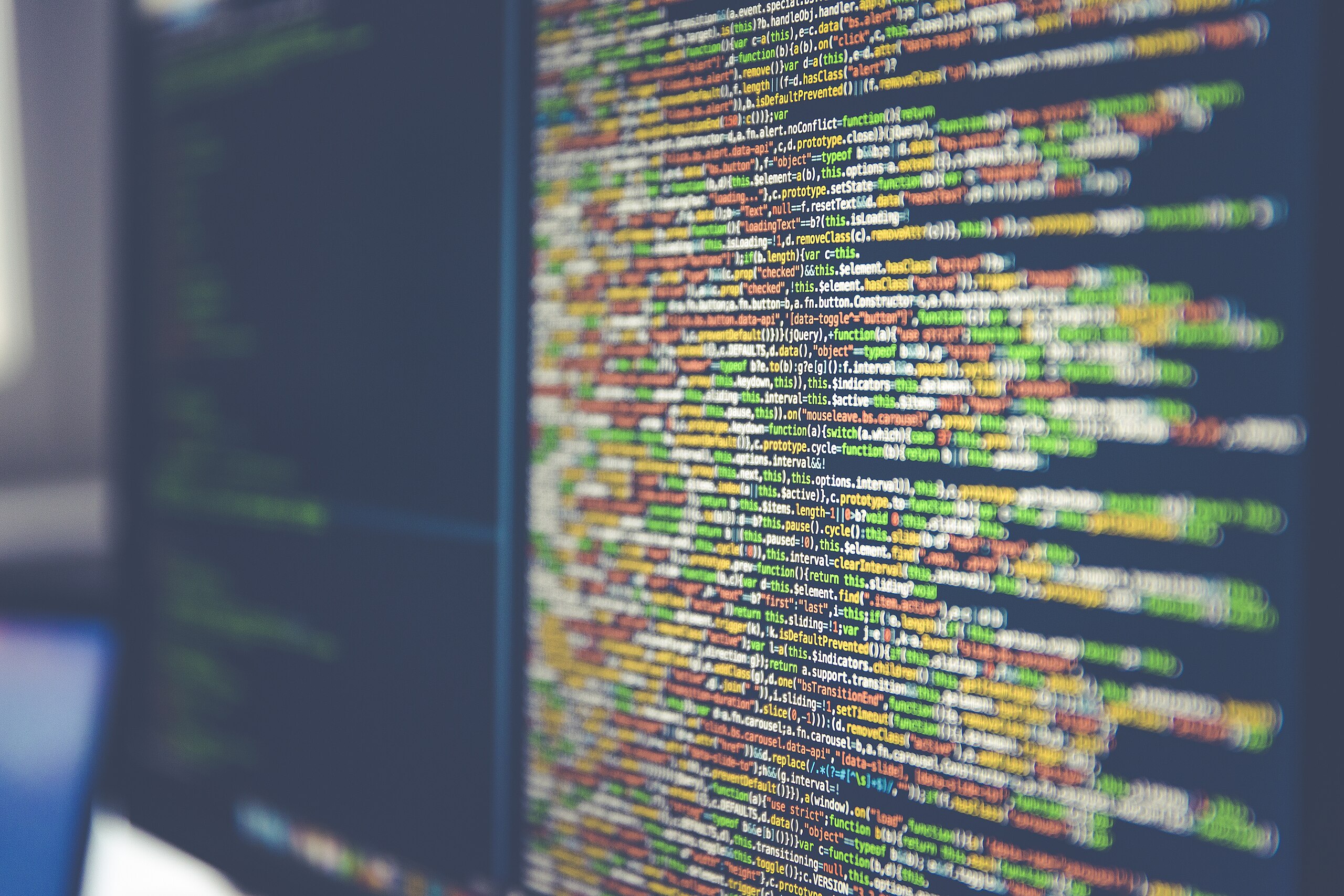
To meet the demand for embedded software engineers, there are several steps you can take to enter this field. One important step is to acquire Linux training. Linux is widely used in the embedded systems industry, so having a strong understanding of Linux can greatly enhance your career prospects. Additionally, gaining knowledge in programming languages such as C and C++ is essential for embedded software engineering. These languages are commonly used in the development of embedded systems and firmware.
To further enhance your skills, consider gaining hands-on experience with microcontrollers and other hardware components commonly used in embedded systems. This can be done through projects or internships that involve working with microcontrollers, general-purpose input/output (GPIO), and other embedded system components.
Moreover, staying updated with industry trends and advancements, such as the Internet of Things (IoT) and wearable technology, can give you an edge in the job market.
Gaining an Edge in the Job Market through Hands-On Experience
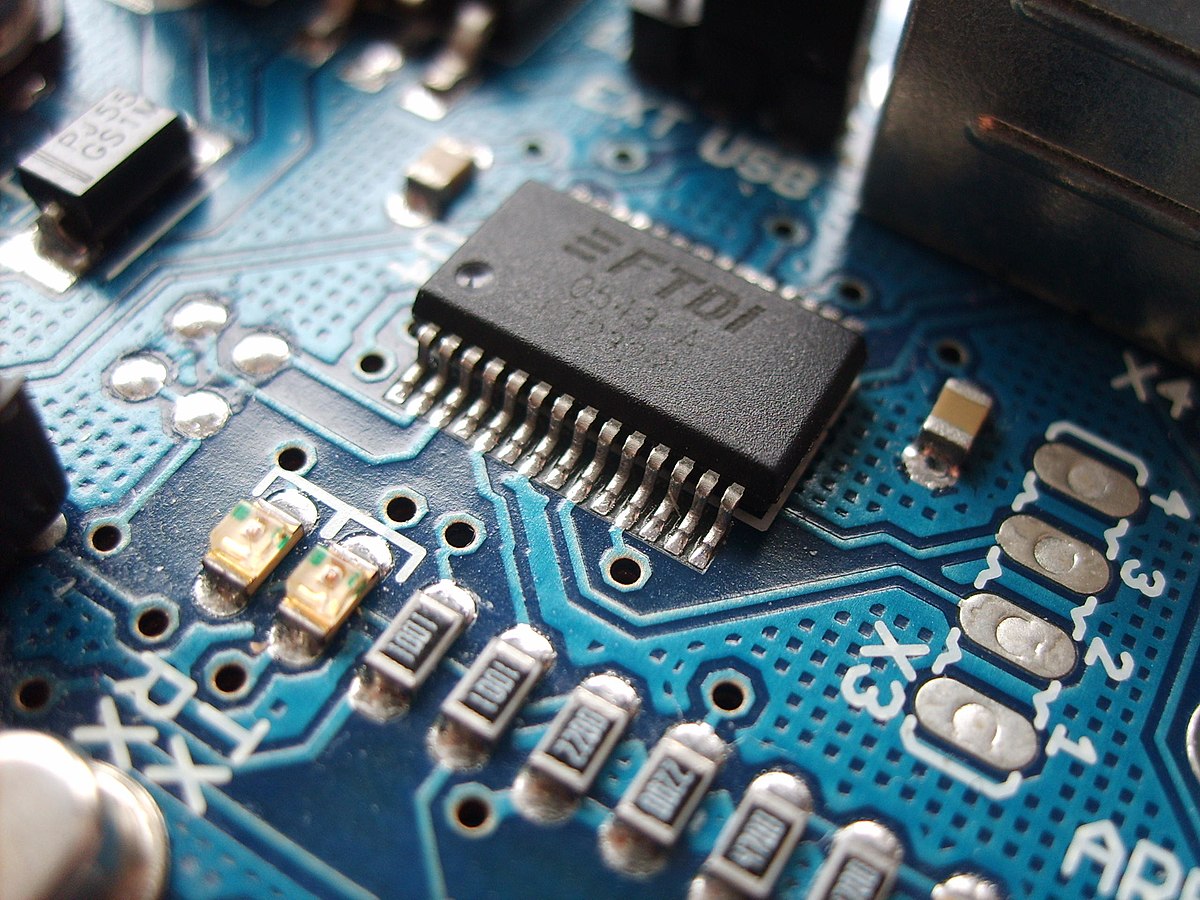
One of the best ways to stand out in the job market as an embedded software engineer is to gain hands-on experience. This can be achieved through Linux training, which provides a solid foundation for working with embedded systems.
Linux is widely used in the embedded software industry, making it essential for aspiring engineers to have a strong understanding of this operating system. By learning Linux, you will be equipped with the necessary skills to work with microcontrollers, develop firmware, and program general-purpose input/output.
Hands-on experience with Linux will also give you an advantage in the growing field of Internet of Things (IoT) and wearable technology. These areas require embedded software engineers who can develop reliable and efficient applications for various devices.
In addition to Linux training, it is important to have a strong understanding of programming languages such as C and C++. These languages are commonly used in embedded software development and will enable you to write efficient and reliable code.
By gaining hands-on experience and acquiring the necessary skills, you will position yourself as a highly valuable candidate in the job market. Employers are constantly seeking embedded software engineers who can bring innovation and expertise to their companies.
So, if you want to gain an edge in the job market and pursue a successful career as an embedded software engineer, consider taking Linux training and honing your programming skills. With the right knowledge and experience, you will be well-equipped to tackle the challenges of this exciting field.
Learning the Fundamentals: ‘C’ Programming
Learning ‘C’ programming is essential for becoming an embedded software engineer. ‘C’ is a powerful and widely used programming language in the field of embedded systems. It provides low-level control and efficient memory management, making it ideal for programming microcontrollers and microprocessors.
By mastering ‘C’, you will gain the skills to write efficient and reliable code for embedded systems, such as medical devices, robotics, and IoT devices. Understanding concepts like interrupts, input/output, and general-purpose input/output (GPIO) will be crucial for your success as an embedded software engineer.
Learning ‘C’ programming will also open doors to various career opportunities, from working at established tech companies like Intel and Amazon to joining exciting startup companies in the embedded systems industry.
To get started with learning ‘C’ programming, you can refer to the book “The C Programming Language” by Brian Kernighan and Dennis Ritchie, the creators of ‘C’. There are also numerous online resources and tutorials available that can help you grasp the fundamentals and practice your skills.
Taking Linux training courses will complement your ‘C’ programming knowledge, as Linux is widely used in embedded systems development. Understanding Linux will enable you to work with bootloaders, serial ports, and other essential components of embedded systems.
Starting with an 8 Bit Micro Controller
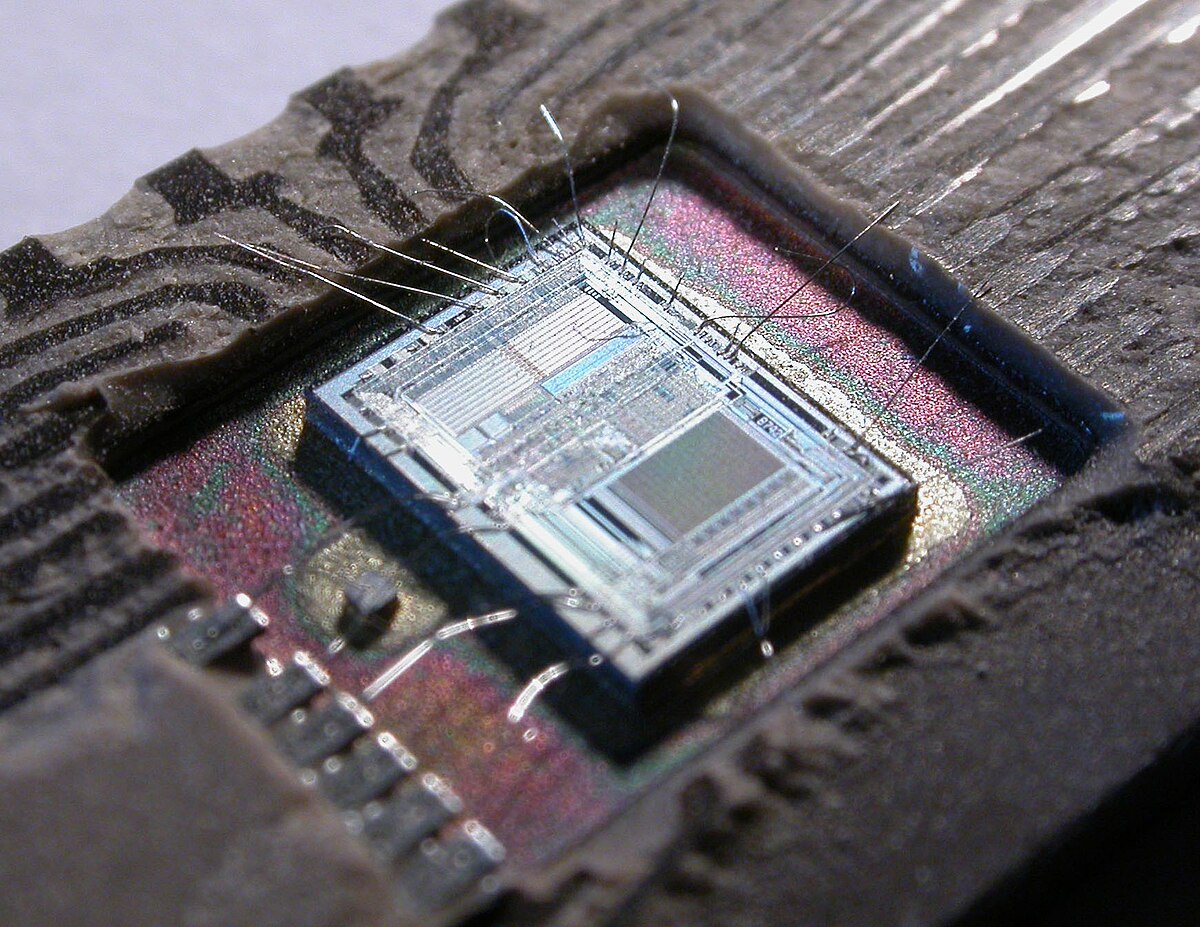
To become an Embedded Software Engineer, start by familiarizing yourself with an 8 Bit Micro Controller. This is a key component of embedded systems and serves as the brain of the device. Learn about its architecture and how it interacts with other hardware components such as general-purpose input/output pins.
Next, dive into programming. The C programming language is widely used in embedded software development, so mastering it is crucial. Practice writing code to control the microcontroller and handle various tasks such as reading input from sensors or controlling output devices.
Additionally, gain knowledge in areas like interrupt handling, real-time computing, and reliability engineering.
Consider taking Linux training as this operating system is commonly used in embedded systems. Understanding Linux will open up more opportunities for you in the field.
Finally, keep up with the latest advancements in embedded software development by following industry blogs, attending workshops, and joining online communities. Stay curious and always be willing to learn and adapt to new technologies.
Mastering Interfacing and Creating Bread Board Circuits
Mastering interfacing and creating breadboard circuits is an essential skill for aspiring embedded software engineers. These skills allow you to connect and control various components and devices, such as microcontrollers, using the breadboard as a prototyping platform.
To become proficient in this area, it is recommended to start with a strong foundation in computer engineering and programming. Understanding the basics of microcontrollers, general-purpose input/output, and embedded systems is crucial. Additionally, knowledge of programming languages like C and C++ is essential for writing the software that will interact with the hardware.
Practical experience is key in mastering interfacing and creating breadboard circuits. Experiment with different components and devices, and learn how to use tools like oscilloscopes, multimeters, and analog-to-digital converters to measure and analyze signals. Additionally, familiarize yourself with serial ports and bootloaders to facilitate communication between the microcontroller and the computer.
Stay updated with the latest advancements in the field by following industry leaders and staying active in online communities. Consider joining forums or participating in open-source projects to enhance your skills and gain practical experience. Remember, practice makes perfect, so dedicate time to hands-on projects and troubleshooting software bugs.
By mastering interfacing and creating breadboard circuits, you will be well-equipped to tackle complex embedded software engineering projects and pursue a successful career in this field.
Exploring the Field of Embedded Systems Engineering
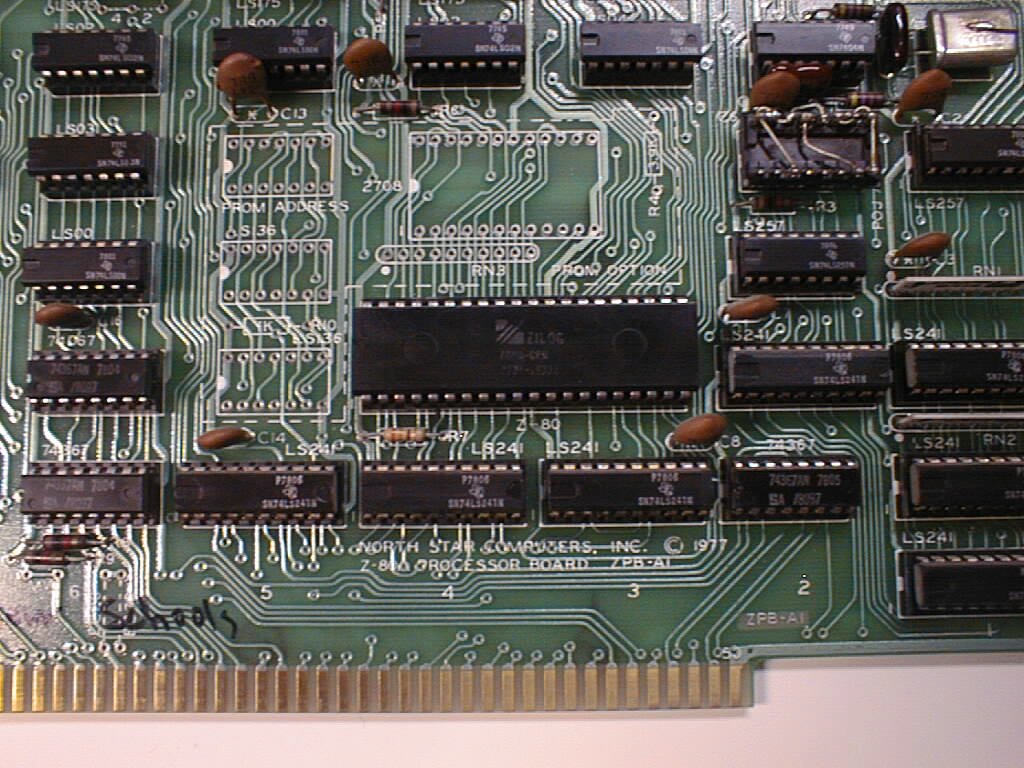
To become an embedded software engineer, it is important to have a strong foundation in Linux training. Linux is widely used in the field of embedded systems engineering and having expertise in this operating system will give you a competitive edge.
Embedded systems are everywhere, from medical devices to electric bicycles, and as an embedded software engineer, you will be responsible for designing and developing the software that controls these systems. This requires a deep understanding of computer hardware and programming languages such as C and C++.
In addition to Linux training, it is also beneficial to have knowledge of other relevant technologies such as real-time computing, general-purpose input/output (GPIO), and human-computer interaction (HCI). Familiarity with tools like oscilloscopes, multimeters, and analog-to-digital converters can also be helpful in troubleshooting and debugging.
To enhance your skills and stay up-to-date with the latest developments in embedded systems engineering, consider joining online communities, attending conferences, and participating in open-source projects. These opportunities will not only expand your knowledge but also connect you with professionals in the field.
Career Path and Interview Tips for Embedded Systems Engineers
If you’re interested in becoming an embedded software engineer, there are several steps you can take to kickstart your career. One essential step is to gain Linux training, as it is widely used in the embedded systems industry. Linux proficiency will enhance your understanding of the operating system and its applications in embedded systems.
In addition to Linux training, it’s crucial to have a strong foundation in computer programming languages such as C and C++. These languages are commonly used in embedded systems development. Familiarize yourself with concepts like general-purpose input/output, interrupts, and human-computer interaction to excel in this field.
To showcase your skills to potential employers, consider working on projects that involve embedded systems. Start with small-scale projects and gradually increase their complexity. This hands-on experience will demonstrate your practical knowledge and problem-solving abilities.
Networking is also essential in this industry. Attend industry conferences, join online communities, and connect with professionals already working in embedded systems engineering. Their insights and experiences can provide valuable guidance and open doors for career opportunities.
When preparing for interviews, make sure to brush up on your knowledge of embedded systems architecture, computer hardware, and microprocessors. Be prepared to discuss your past projects and any software bugs you encountered and how you resolved them. Emphasize your ability to work in a team and your attention to detail, which are crucial skills for embedded systems engineers.
Consider seeking opportunities with startup companies or organizations involved in innovative technologies. These environments often offer more opportunities for growth and hands-on experience.